C++ Reference Vs Pointer: A Deep Dive Into The Core Differences
Hey there, fellow coders! If you're diving into the world of C++ programming, you've probably stumbled upon two crucial concepts: references and pointers. These bad boys are essential when it comes to memory management and data manipulation. But what exactly are they, and how do they differ? Stick around, because we're about to break it down for you in a way that's both informative and easy to digest. Let's jump right in, shall we?
C++ reference vs pointer is a topic that can get pretty tricky if you don't have the right guidance. Imagine trying to navigate a maze without a map—yeah, it’s kinda like that. Both references and pointers are used to indirectly access data, but they behave in fundamentally different ways. Understanding these differences is key to writing clean, efficient, and bug-free code. So, buckle up, because this ride is gonna be worth it!
Before we dive deeper, let me just say that mastering references and pointers isn't just about knowing the syntax—it's about understanding the nuances and knowing when to use which tool. By the end of this article, you'll not only know the technical differences but also how to apply them in real-world scenarios. Ready to level up your C++ game? Let's go!
- Unlocking The Power Of Rank Checker Api For Seo Success
- Unlocking The Secrets Of Free Website Rank A Comprehensive Guide
What is a Reference in C++?
A reference in C++ is like an alias for a variable. Think of it as giving your best friend a nickname—it's still the same person, just with a different name. When you declare a reference, you're essentially creating another name for an existing variable. Here's the kicker: once you assign a reference to a variable, you can't change it to refer to something else. It's a one-and-done deal, my friend.
Here's a quick example to illustrate how references work:
int num = 42; // Declare an integer
int &ref = num; // Create a reference to 'num'
ref = 100; // This changes the value of 'num' to 100
- Nikki Catsouras The Tragic Car Accident That Captivated The Internet
- Unlocking The Secrets Of Your Websites Performance With Google Ranking Checker Websites
See how easy that was? References make your code cleaner and more intuitive. Plus, they're safer to use than pointers, as we'll see later on.
What is a Pointer in C++?
Now, let's talk about pointers. A pointer is like a treasure map—it points to the location of a variable in memory. Unlike references, pointers can be reassigned to point to different variables. They're more flexible, but with great power comes great responsibility. If you're not careful, pointers can lead you down a path of null pointer dereferences and memory leaks. Yikes!
Here's how you declare and use a pointer:
int num = 42; // Declare an integer
int *ptr = # // Create a pointer to 'num'
*ptr = 100; // Dereference the pointer to change the value of 'num'
Pointers give you direct access to memory addresses, which can be both a blessing and a curse. Use them wisely, and they'll be your best friend. Abuse them, and they'll turn into a nightmare.
Key Differences Between References and Pointers
Now that we've covered the basics, let's break down the key differences between references and pointers. This is where things get interesting:
1. Initialization
References: Must be initialized when declared and cannot be reassigned later.
Pointers: Can be initialized later and can be reassigned to point to different variables.
2. Null Values
References: Cannot hold null values. They must always refer to a valid variable.
Pointers: Can hold null values, which makes them more versatile but also more dangerous.
3. Memory Address Access
References: Automatically dereference to the value they refer to.
Pointers: Require explicit dereferencing using the * operator to access the value they point to.
4. Syntax and Readability
References: Lead to cleaner and more readable code.
Pointers: Can make code harder to read, especially when dealing with complex operations.
When to Use References vs Pointers
Choosing between references and pointers depends on the specific requirements of your program. Here's a quick guide to help you decide:
- Use references when you need a simple alias for a variable and don't need the flexibility of reassignment.
- Use pointers when you need to dynamically allocate memory or when you need the ability to point to different variables.
- References are great for function parameters where you want to avoid copying large objects.
- Pointers are ideal for implementing data structures like linked lists or trees.
Advantages and Disadvantages
Advantages of References
- Cleaner syntax and easier to read.
- No risk of null references.
- Automatic dereferencing.
Disadvantages of References
- Cannot be reassigned to refer to a different variable.
- Less flexible than pointers.
Advantages of Pointers
- More flexible and versatile.
- Can dynamically allocate memory.
- Can point to different variables.
Disadvantages of Pointers
- More prone to errors like null pointer dereferences.
- Can lead to memory leaks if not managed properly.
- Harder to read and understand.
Common Use Cases
1. Function Parameters
References are often used as function parameters to avoid copying large objects. This improves performance and reduces memory usage.
void modifyValue(int &value) {
value = 100;
}
int num = 42;
modifyValue(num); // Changes the value of 'num' to 100
2. Dynamic Memory Allocation
Pointers are essential for dynamic memory allocation using operators like new
and delete
.
int *ptr = new int(42);
delete ptr; // Free the allocated memory
3. Data Structures
Pointers are commonly used in implementing data structures like linked lists, trees, and graphs.
Best Practices
Here are some best practices to keep in mind when working with references and pointers:
- Prefer references over pointers whenever possible for simplicity and safety.
- Always initialize pointers to avoid dangling pointers.
- Use smart pointers like
std::unique_ptr
andstd::shared_ptr
to manage memory automatically. - Document your code clearly to make it easier for others (and your future self) to understand.
Real-World Examples
Example 1: Using References for Function Parameters
void swap(int &a, int &b) {
int temp = a;
a = b;
b = temp;
}
int x = 10, y = 20;
swap(x, y); // Swaps the values of x and y
Example 2: Using Pointers for Dynamic Memory Allocation
int *createArray(int size) {
return new int[size];
}
int *arr = createArray(10);
delete[] arr; // Free the allocated memory
Conclusion
Alright, folks, that wraps up our deep dive into C++ reference vs pointer. By now, you should have a solid understanding of what they are, how they differ, and when to use each one. Remember, mastering these concepts takes practice, so don't be afraid to experiment with different scenarios in your code.
Before you go, here's a quick recap of the key points:
- References are aliases for variables and must be initialized when declared.
- Pointers are more flexible but require careful management to avoid errors.
- Choose references for simplicity and pointers for versatility.
- Follow best practices to write clean, efficient, and bug-free code.
So, what are you waiting for? Grab your favorite IDE, fire up your compiler, and start coding! And don't forget to drop a comment below if you have any questions or feedback. Happy coding, and see you in the next one!
Table of Contents
- What is a Reference in C++?
- What is a Pointer in C++?
- Key Differences Between References and Pointers
- When to Use References vs Pointers
- Advantages and Disadvantages
- Common Use Cases
- Best Practices
- Real-World Examples
- Conclusion
- Unlocking The Power Of Serp Position Tracker For Your Online Success
- The Ultimate Guide To Using A Keyword Ranking Google Checker
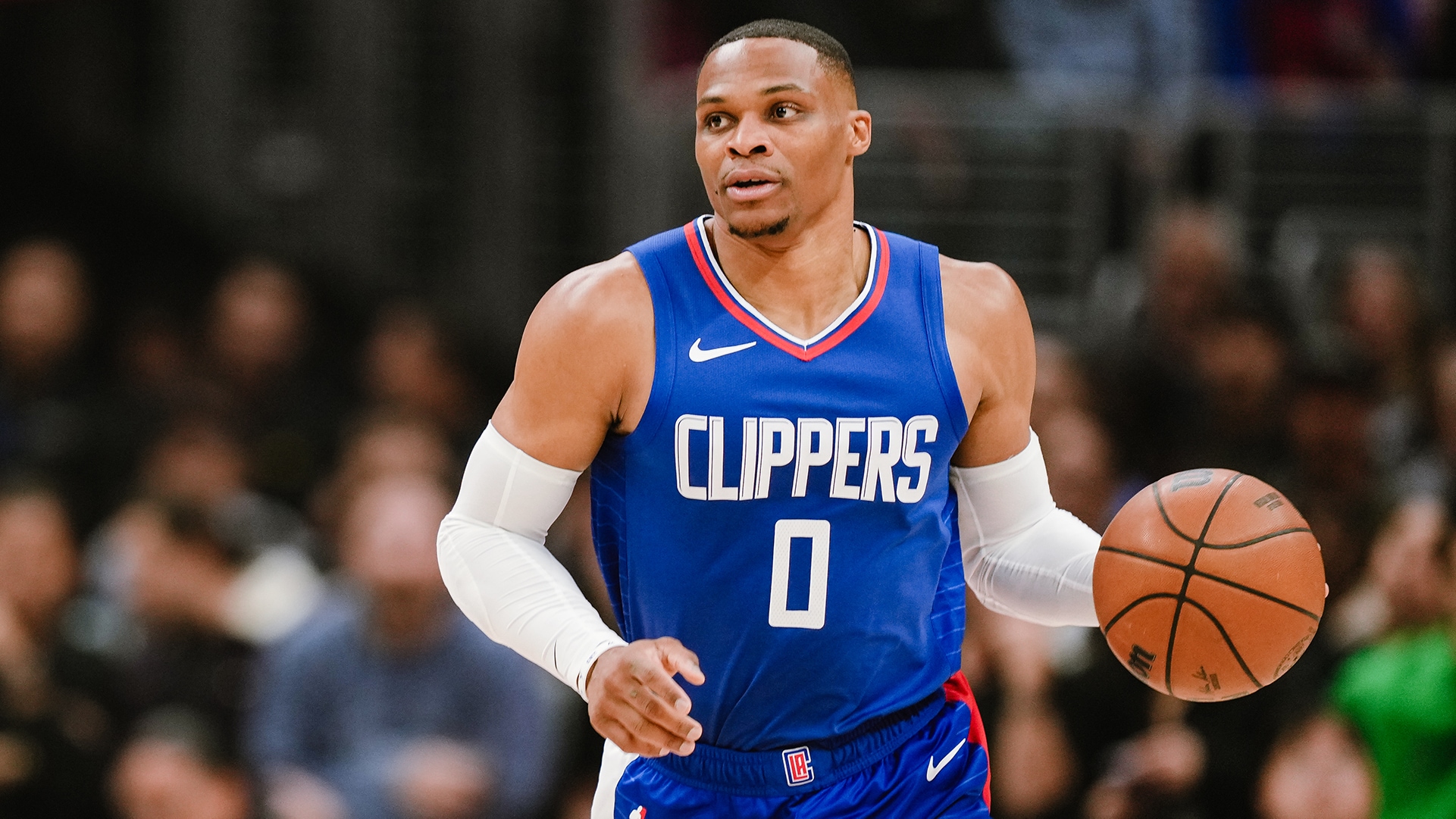
Gallery Clippers vs Denver Nuggets (11.27.23) Photo Gallery

English Pointer vs Boxer Breed Comparison Petzlover

Reference vs drawing Drawings, Historical figures, Historical